a
map object is a layer, it can be transparents and animate
actually just map with tiles of 16*16 or 32*32 can be draw
create
and load
create :
it's very easy : struct map my_map ;
load :
to load a map, you need the bitmap with tiles tab, and the map
array (use mappy
to generate it)
use set_map function to load tiles and array in your map object
* set_map
void set_map(struct map * map,short * map_tab,
unsigned short size_x, unsigned short size_y,
int scrool_x, int scrool_y, unsigned char * tiles, unsigned short
nb_tiles, unsigned char size_tile_x, unsigned char size_tile_y,
unsigned short transp_color)
struct map * map
pointer
to the map object
unsigned short
size_x x
size of the map, in tiles
unsigned short
size_y y
size of the map, in tiles
int scrool_x the
scrool value in x where the map will be init
int scrool_y the
scrool value in y where the map will be init
unsigned char
* tiles the
bitmap where is the tab of tiles
unsigned short
nb_tiles number
of tiles to load
unsigned char
size_tile_x size
of the tile, in x
unsigned char
size_tile_y size
of the tile, in y
unsigned short
transp_color transparent color (0 = no
transparent tiles)
exemple :

|
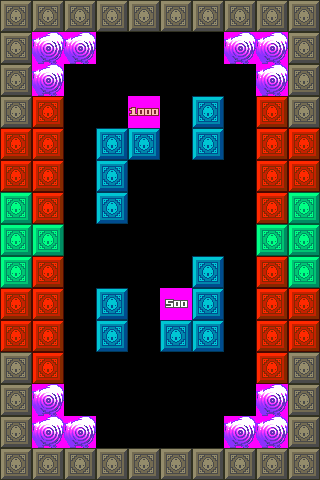
|
short my_map[15][10] = {
{ 1, 1, 1, 1, 1, 1, 1, 1, 1, 1 },
{ 1, 12, 12, 0, 0, 0, 0, 12, 12, 1 },
{ 1, 12, 0, 0, 0, 0, 0, 0, 12, 1 },
{ 1, 4, 0, 0, 25, 0, 2, 0, 4, 1 },
{ 4, 4, 0, 2, 2, 0, 2, 0, 4, 4 },
{ 4, 4, 0, 2, 0, 0, 0, 0, 4, 4 },
{ 3, 4, 0, 2, 0, 0, 0, 0, 4, 3 },
{ 3, 3, 0, 0, 0, 0, 0, 0, 3, 3 },
{ 3, 4, 0, 0, 0, 0, 2, 0, 4, 3 },
{ 4, 4, 0, 2, 0, 24, 2, 0, 4, 4 },
{ 4, 4, 0, 2, 0, 2, 2, 0, 4, 4 },
{ 1, 4, 0, 0, 0, 0, 0, 0, 4, 1 },
{ 1, 12, 0, 0, 0, 0, 0, 0, 12, 1 },
{ 1, 12, 12, 0, 0, 0, 0, 12, 12, 1 },
{ 1, 1, 1, 1, 1, 1, 1, 1, 1, 1 }
};
|
struct
map bg ; //
declare the map
set_map(&bg,(short
*)my_map,10,15,0,0,(unsigned char *)tile_bitmap,26,32,32,0xB3)
; // load
the map
|
caution, tile number 0 will never be draw, so use it for complette
transparent tiles
set_map function will scans all tiles to search for transp color,
to draw thems with bitblt or transpbitblt
if all yours tiles are not transparents, put 0 at transp color,
and tiles will not be scan and will be draw in bitblt
so, you can't have color 0 for transparencie color in your bmp,
that the same way with gfx object
with set_map you can't load animated tiles, to load it use load_animated_tile
* load_animated_tile
load_animated_tile load and init an animated tile in a map object
void load_animated_tile(struct map * map, unsigned
char * tile_bmp, unsigned short nb_tiles, unsigned short id_tile,
unsigned char type_anim, unsigned short time_anim)
struct map * map pointer
to the map object
unsigned char
* tile_bmp pointeur image where is
animated tile
unsigned char
nb_tiles number
of frame of the animated tile
char id_tile the
number in tab tile of the map object where load the animated tile
unsigned char
type_anim type of anim for
this tile ( 0 : 123123... 1 : 123333... 2 : 123111...
3 : 1232123.. )
unsigned short
time_anim time in between 2 frames of
the anim
exemple :
you got a map and the tile number 78, flower, got this anim :
animate in 123123 with 150ms between every frames,
3 16*16 frames
load_animated_tile(&my_map,(unsigned
char *)tile_78,3,78,0,150) ;
map draw functions
* draw_map_animated_16
this function draw on the entire screen
an animated map, tile size must be 16*16
the part of the map will be draw depend
of scrool_x and scrool_y map object properties
void draw_map_animated_16(struct
map * map)
struct map * map
pointer to the map object
to draw
* draw_map_animated_32
this function draw on the entire screen
an animated map, tile size must be 32*32
the part of the map will be draw depend
of scrool_x and scrool_y map object properties
void draw_map_animated_32(struct
map * map)
struct map * map
pointer to the map object
to draw
exemple : draw_map_animated_16(&bg) ;
map scrool functions
map object got 4 properties, 2 var who define scrool value and
2 other who are scrool max value
scrool_x : actual scroll in x value, 0 : left
scrool_y : actual scroll in y value, 0 : up
map_max_x : the max value for the x scrool, is compute on set_map
map_max_y : the max value for the y scrool, is compute on set_map
so 0 <= scrool_x <= map_max_x and
0 <= scrool_y <=
map_max_y
acces to this propertie :
struct map my_map ; //
declare the map object 'my_map'
my_map.scrool_x += 50 ; // increse the scrool_x
of my_map of 50 pixels
my_map.scrool_y = 2986 ; // define my_map scrool_y
at 2986 pixel from the up of the map
...
* auto_scrool
this function auto scrool a map to folow a rect
void auto_scrool(struct map * map, short pos_x,
short pos_y, short size_x, short size_y, short limit_up, short
limit_down, short limit_right, short limit_left)
struct map * map pointer
to the map
short pos_x pos_x
of the rect to folow
short pos_y pos_y
of the rect to folow
short size_x x
size of the rect
short size_y y
size of the rect
short limit_up limit
up on from the up of the screen where be the rect to scrool
short limit_down limit
down ...
short limit_right limit
right ...
short limit_left limit
left ...
exemple : auto_scrool(&bg, link.pos_x>>1,
link.pos_y>>1, 16, 24, 60, 80, 80) ;
* folow_gfx
this function use auto_scrool to folow an gfx object
the gfx object must have pos_x pos_y propertie define on the map
void folow_gfx(struct map * map, struct gfx
* gfx, short limit_up, short limit_down, short limit_right, short
limit_left)
struct
map * map pointer
to the map
struct gfx * gfx
pointer
to the gfx object
short limit_up limit
up on from the up of the screen where be the gfxobject for scrool
short limit_down limit
down ...
short limit_right limit
right ...
short limit_left limit
left ...
exemple : folow_gfx(&level, &link, 60,
60, 80, 80) ;
tiles detection
all tiles got a 'walkable' properties
it's an unsigned char, so you got 8 bit by tile to define his
detection
bite
7 -> masq = 10000000 = 128 = 0x80
bite
6 -> masq = 01000000 = 64 = 0x40
bite
5 -> masq = 00100000 = 32 = 0x20
bite
4 -> masq = 00010000 = 16 = 0x10
bite
3 -> masq = 00001000 = 8 = 0x8
bite
2 -> masq = 00000100 = 4 = 0x4
bite
1 -> masq = 00000010 = 2 = 0x2
bite
0 -> masq = 00000001 = 1 = 0x1
you need to make your own protocol colision
ex :
bite 0 define if tile is block when go up
bite 1 ............................................down
bite 2 ............................................left
bite 3 ............................................right
bite 4 define is tile can be broken
ect ...
* set_walkable_on_tiles
this function define walkable propertie of tiles
void set_walkable_on_tiles(struct map * map,unsigned
char * walkable, unsigned short nb_tiles)
struct map * map pointer
to the map
unsigned char * walkable
pointer to the tab containing all walkable var
unsigned short nb_tiles
the number of tile to
define
exemple :
set_walkable_on_tiles(&level,(unsigned
char *)"01111111111100000000000000",26) ;
unsignedchar tile_colision[50] = { 0,0x4,0,0xF,0xF,0xF,0xF,0xF,0xF,0x4,
0,0x4,0x4,0,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,0xF,
0xF,0xF,0xF,0xF,0xF,0xF,0,0,0,0,0,0,0 };
set_walkable_on_tiles(&game,tile_colision,42);
* get_walkable
this function return the walkable var
of the tile number 'id' of the map 'map'
char get_walkable(struct map * map, short id)
* set_walkable
this function set the walkable var of
the tile number 'id' of the map 'map' with 'walkable' value
void set_walkable(struct map * map, short id,
unsigned char walkable)
* test_tile
this function return the mask between
the walkable var of the tile number 'id' of the map 'map' and
the specified value 'mask'
char test_tile(struct map * map, short id_tile,
unsigned char masq)
by exemple if you define bite 3 to block
down, test tile with 0x8, if the return value is != 0, your tile
will block down else, you can move down
* get_tile
this function return the tile number display
at the x,y screen coordonate
short get_tile(struct map * map, short x, short
y)
* set_tile
this function change the tile display
at the x,y screen coordonate by the specified tile 'id'
void set_tile(struct map * map, short x, short
y, short id)
* get_start_pos_of_a_tile
this function return the pos_x pos_y map
position (remove scrool value to the pos compute to get the tile
screen pos) of the tile display at the specified screen x,y coordonate
void get_start_pos_of_a_tile(struct map * map,unsigned
short x,unsigned short y,short *startx,short *starty)
struct map * map pointer
to the map
unsigned short x screen
x pos where is display the tile to get coordonate
unsigned short y screen
y pos where is display the tile to get coordonate
short * startx pointer
to the var who'll receve the value of the pos_x of the tile (pos
on map)
short * starty pointer
to the var who'll receve the value of the pos_y of the tile (pos
on map)
concretely use tile detection :
you got a gfx object nammed 'mario' (with his
pos define on the map nammed 'game')
exemple of code to detect and move mario on the left : (here,
tile protocol define bite 1 (0x2) to block on the left)
// function to test tiles display at the left of mario (here we
test 3 point to the left of mario : up left, middle left and down
left of mario. number of point to test depend of your sprite and
tile size)
char can_mario_left(void)
{
if(mario.pos_x
<= 0) return 0 ;
if(!
test_tile(&game,get_tile(&game,mario.pos_x - game.scrool_x,mario.pos_y+1
- game.scrool_y),0x2) //
test up left of mario
if(!
test_tile(&game,get_tile(&game,mario.pos_x - game.scrool_x,mario.pos_y
+ 14 - game.scrool_y),0x2)) // test middle left
of mario
if(!
test_tile(&game,get_tile(&game,mario.pos_x - game.scrool_x,mario.pos_y
+ mario.size_y-1 - game.scrool_y),0x2)) // test
down left of mario
return
1 ; // if the 3 point are not block on left, return 1
return 0 ; //
if a blocking tile detected, return 0
}
// this function test if mario can move and if can, move
him
unsigned short go_left(unsigned char powa)
{
do
{
if(can_mario_left()) mario.pos_x--
;
else
return
0 ;
} while(powa--)
;
return 1 ;
}
|